FORMVIEW
FormView is a new data-bound control that is nothing but a templated version of DetailsView control.
The major difference between DetailsView and FormView is, here user need to define the rendering template for each item.
Use the FormView control to:
Display
Insert
Edit
Delete
Page
Database records
The formView control is completely template driven
Html Coding for GridView
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False">
<Columns>
<asp:BoundField DataField="Id" HeaderText="ID" />
<asp:BoundField DataField="name" HeaderText="Name" />
<asp:HyperLinkField DataNavigateUrlFields="Id"
DataNavigateUrlFormatString="Details.aspx?Id={0}"
HeaderText="Details" Text="Select" />
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
Html Coding FormView
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head2" runat="server">
<title></title>
</head>
<body>
<form id="form2" runat="server">
<div>
<asp:FormView ID="FormView1" runat="server"
DataKeyNames="Id"
DataSourceID="SqlDataSource1" BackColor="Gray" Width="200px">
<EditItemTemplate>
Id:
<asp:Label ID="IdLabel1" runat="server"
Text='<%# Eval("Id") %>' />
<br />
name:
<asp:TextBox ID="nameTextBox" runat="server"
Text='<%# Bind("name") %>' />
<br />
age:
<asp:TextBox ID="ageTextBox" runat="server"
Text='<%# Bind("age") %>' />
<br />
mobile:
<asp:TextBox ID="mobileTextBox" runat="server"
Text='<%# Bind("mobile") %>' />
<br />
address:
<asp:TextBox ID="addressTextBox" runat="server"
Text='<%# Bind("address") %>' />
<br />
<asp:LinkButton ID="UpdateButton" runat="server"
CausesValidation="True" CommandName="Update" Text="Update" />
<asp:LinkButton ID="UpdateCancelButton"
runat="server" CausesValidation="False"
CommandName="Cancel" Text="Cancel" />
</EditItemTemplate>
<InsertItemTemplate>
name:
<asp:TextBox ID="nameTextBox" runat="server" Text='<%# Bind("name") %>' />
<br />
age:
<asp:TextBox ID="ageTextBox" runat="server"
Text='<%# Bind("age") %>' />
<br />
mobile:
<asp:TextBox ID="mobileTextBox" runat="server"
Text='<%# Bind("mobile") %>' />
<br />
address:
<asp:TextBox ID="addressTextBox" runat="server"
Text='<%# Bind("address") %>' />
<br />
<asp:LinkButton ID="InsertButton" runat="server"
CausesValidation="True" CommandName="Insert"
Text="Insert" />
<asp:LinkButton ID="InsertCancelButton"
runat="server" CausesValidation="False"
CommandName="Cancel" Text="Cancel" />
</InsertItemTemplate>
<ItemTemplate>
Id:
<asp:Label ID="IdLabel" runat="server"
Text='<%# Eval("Id") %>' />
<br />
name:
<asp:Label ID="nameLabel" runat="server"
Text='<%# Bind("name") %>' />
<br />
age:
<asp:Label ID="ageLabel" runat="server"
Text='<%# Bind("age") %>' />
<br />
mobile:
<asp:Label ID="mobileLabel" runat="server"
Text='<%# Bind("mobile") %>' />
<br />
address:
<asp:Label ID="addressLabel" runat="server"
Text='<%# Bind("address") %>' />
<br />
</ItemTemplate>
</asp:FormView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:dbcon %>"
SelectCommand="SELECT * FROM [reg] WHERE ([Id] = @Id)">
<SelectParameters>
<asp:QueryStringParameter Name="Id"
QueryStringField="Id" Type="Int32" />
</SelectParameters>
</asp:SqlDataSource>
</div>
</form>
</body>
</html>
C# Coding
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
public partial class Formviewss : System.Web.UI.Page
{
SqlConnection con;
SqlCommand cmd;
SqlDataAdapter adp;
SqlDataReader rd;
DataSet ds;
string query;
public void dbcon()
{
string connn = (System.Configuration.ConfigurationManager.ConnectionStrings["dbcon"].ToString());
con = new SqlConnection(connn);
con.Open();
}
protected void Page_Load(object sender, EventArgs e)
{
dbcon();
query = "select * from reg";
cmd = new SqlCommand(query, con);
adp = new SqlDataAdapter(cmd);
ds = new DataSet();
adp.Fill(ds);
rd = cmd.ExecuteReader();
if (ds.Tables[0].Rows.Count > 0)
{
GridView1.DataSource = ds;
GridView1.DataBind();
}
else
{
ds.Tables[0].Rows.Add(ds.Tables[0].NewRow());
GridView1.DataSource = ds;
GridView1.DataBind();
int columncount = GridView1.Rows[0].Cells.Count;
GridView1.Rows[0].Cells.Clear();
GridView1.Rows[0].Cells.Add(new TableCell());
GridView1.Rows[0].Cells[0].ColumnSpan = columncount;
GridView1.Rows[0].Cells[0].Text = "No Records Found";
}
}
}
First - Add New WebForm - Select GridView from Data ToolBox
Next - Add Database from Solution Explorer - Create Table Name - Right Click Table - Select Add New Table
Add - the Required Column Fields Name - Id Set Primary Key
Select - Id (Is Identity True) & Field Name Add name,age,mobile,address - Table Name (reg)
Next - Save Table - Select Update Button
Next - Select Update Database - Save the Table
Add the Table Name & Table Fields in Database
Right click - Id Set the Primary Key
Next - Select Gridview - Right Click - Select Edit Column
Add - Select Two BoundField -Add - Remove Auto Generate Columns
Next - Change BoundField Name HeaderText =ID
Next - Add DataField Name - For Database Field Name Id For Bind Data
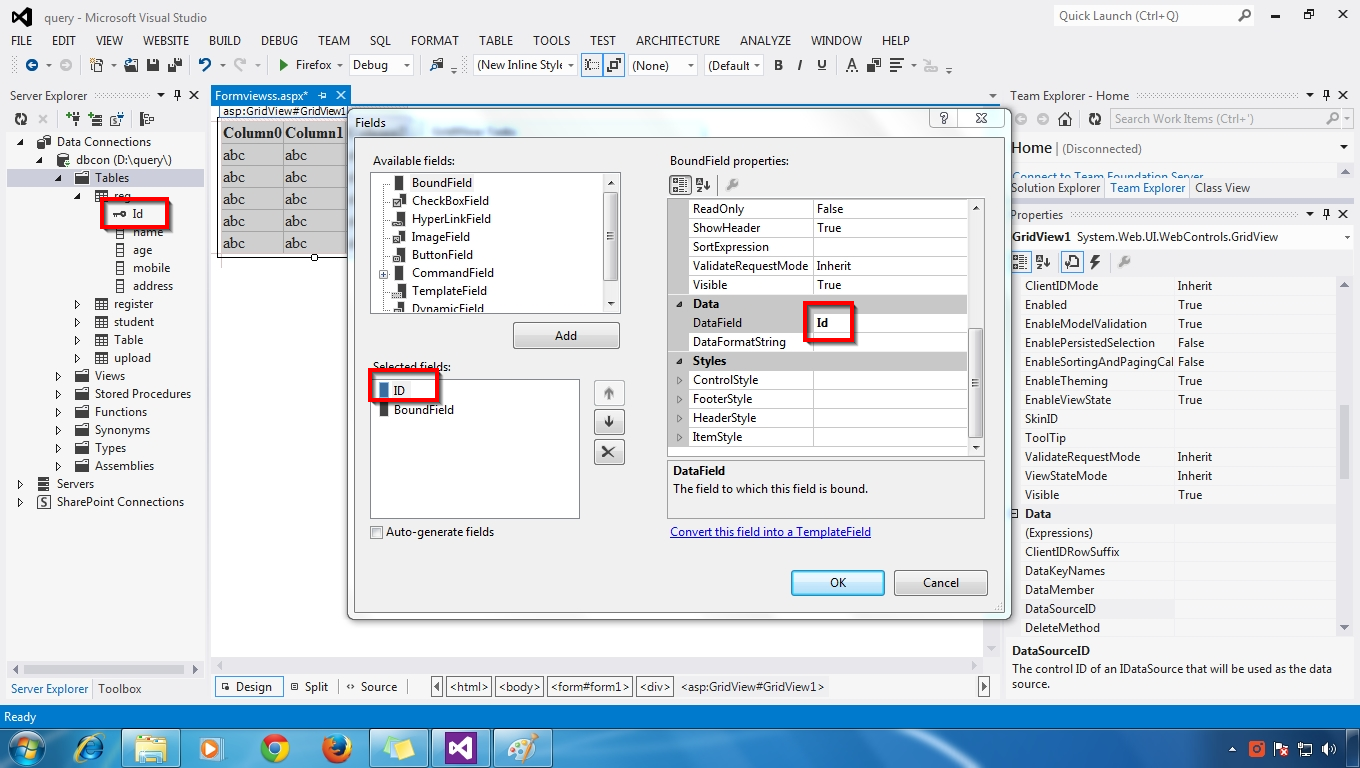
Next - Change HeaderText = Name for Display
Next - DataField Change database Field Name - name
Next - Add - HyperLinkField - Change HeaderText - Name - Details
Next - Change the name - Header Text = Details & Text = Select & DataNavigationfirlds = Id & DataNavigationurlformatString = Details.sapx?Id={0} - Click Ok Button
Databound to GridView
Next - Insert values to reg Table
Next - Select - Details.aspx Form
Select - FormView - RightClick - Select - New DataSource
Next - Pop Window show - Select Database - Ok Button Click
Next - Select - Data Base Name(dbcon) - Next - button Select
Select - Reg Table Name - Select Where Button
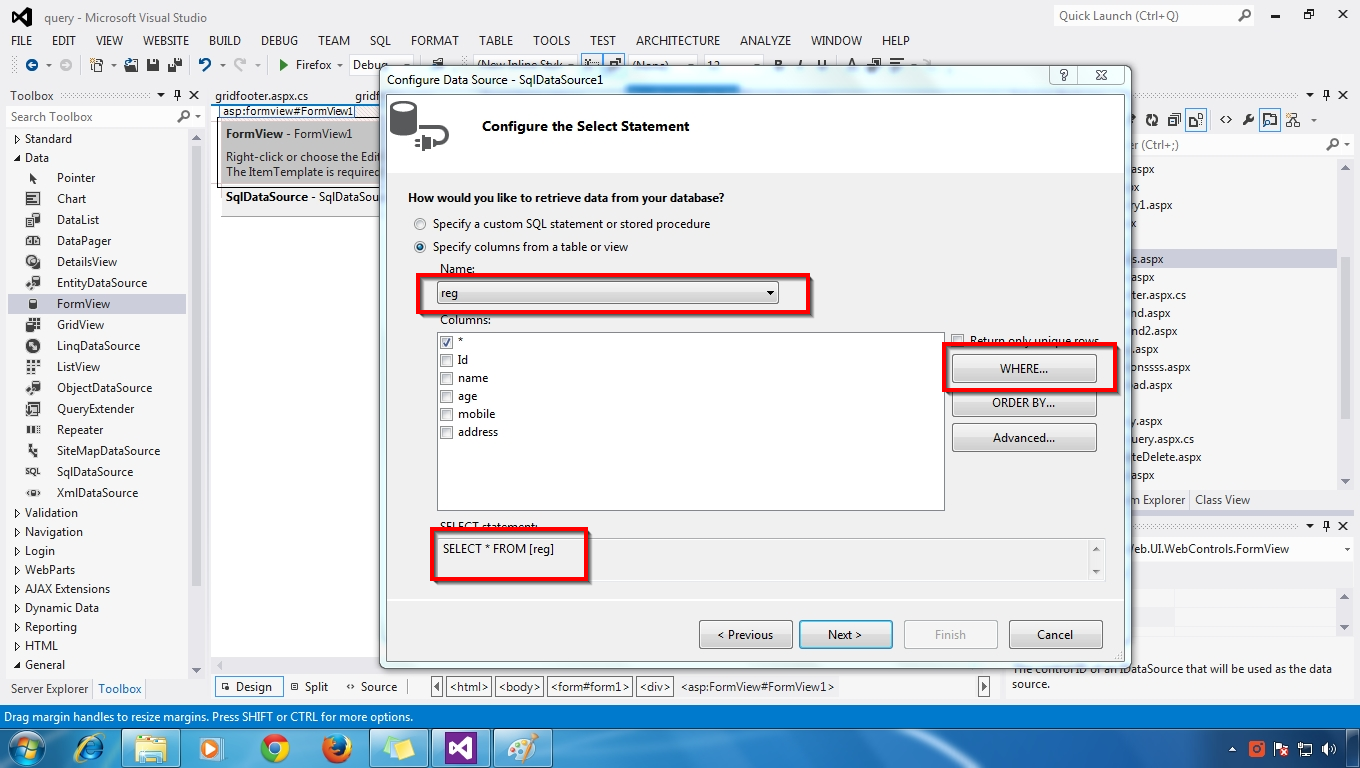
Select - ColumnName = Id & Operatopr = & Source= QueryString & QueryString field -Id - Add Button Click
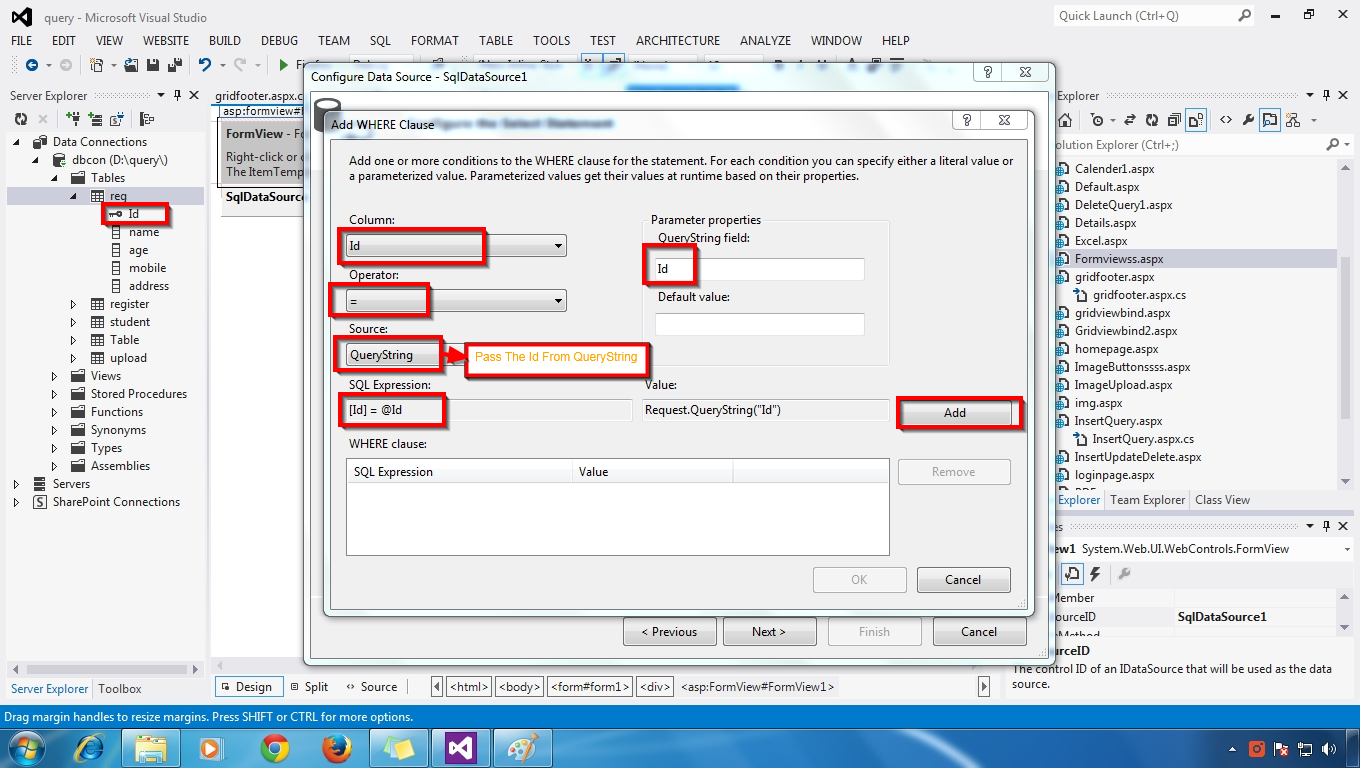
Sql Expression Generate - Click - OK Buttton
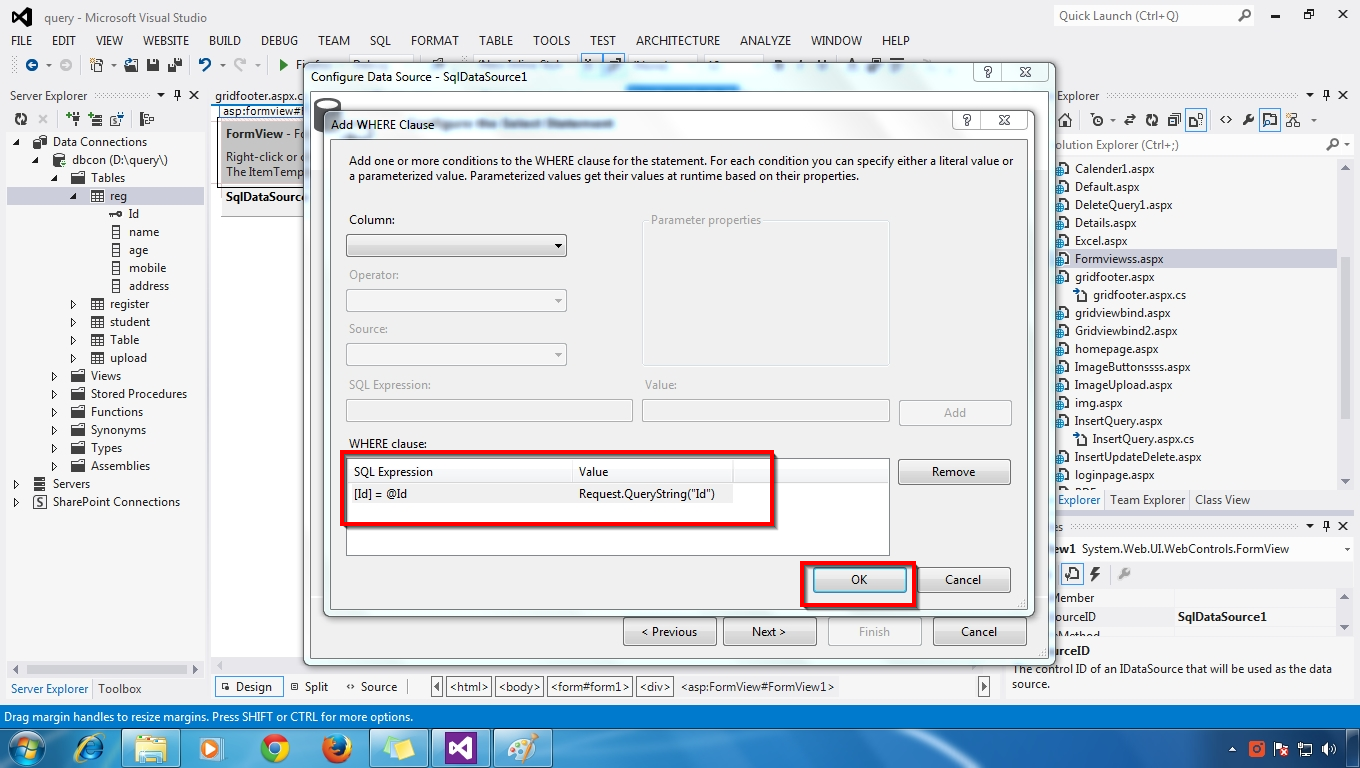
Next - Select Query with Where Conditions Generates - Click Next Button
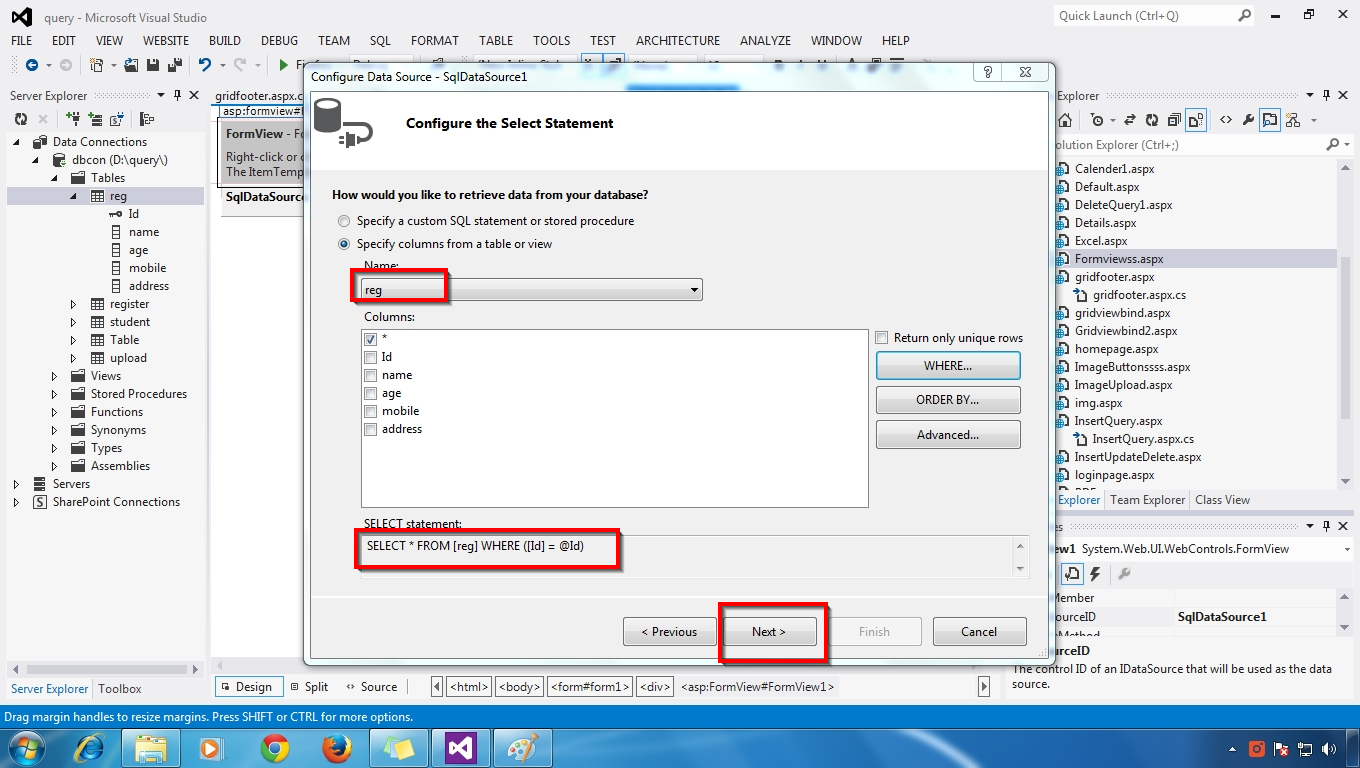
Next - Test query - View reg table Selected - Select Query Generated - Finish Button Click
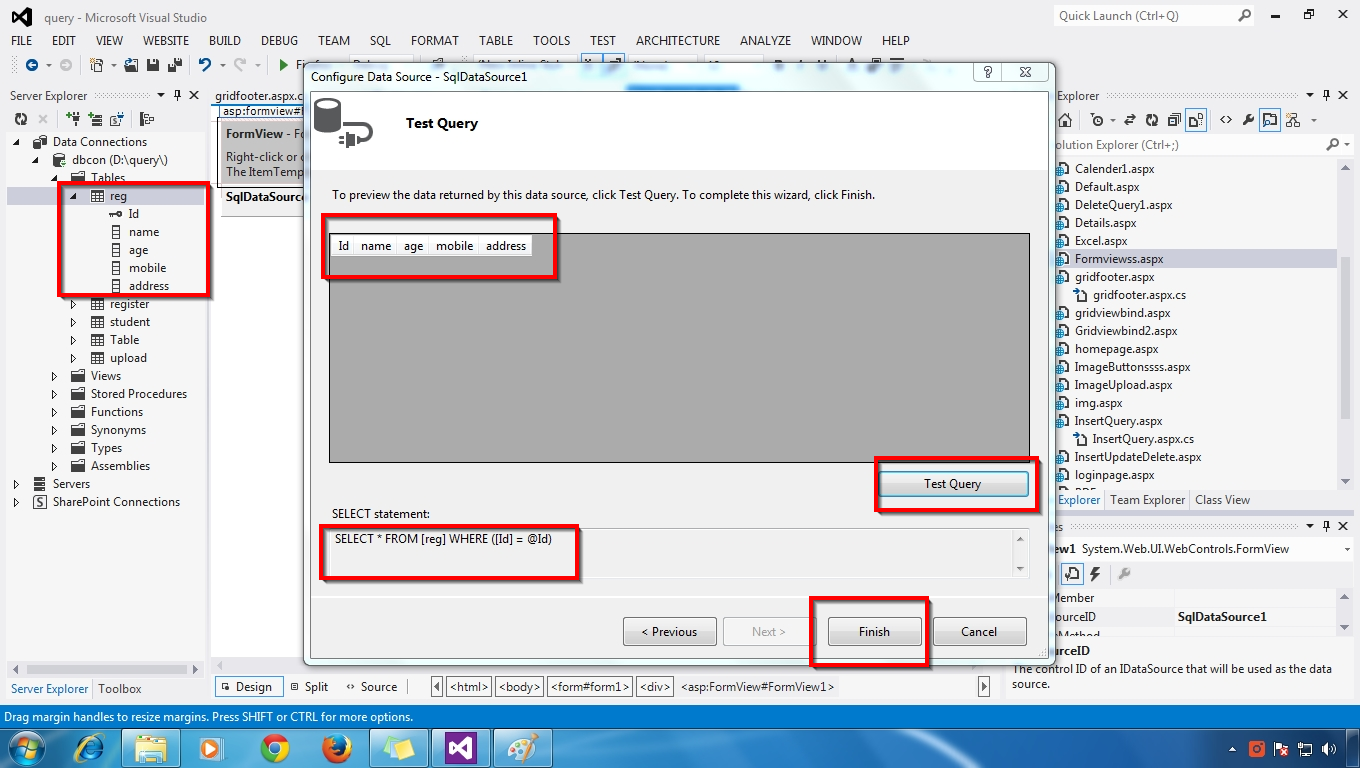
Next - Databoud to FormView
Query StringFields Added Id
Form View Added DataKeyNames = Id
Next - Go to Coding Page - Create NameSpaces & Create DataBase Connection
Next - Select Page Load - Write Select Query Display table Values to GridView
Next - Press F5 Button - Show reg table Values - Select Id=1 Values
Display the Id=1 Values in FormView
Next - Select Id =2 Values Diaplay Detail in FormView
try the Delete Command
0 comments:
Post a Comment